Set up an http server in node in 3 steps
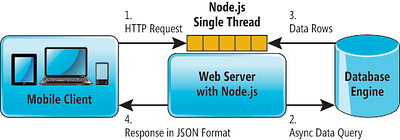
Setup an HTTP Server in Node.js in 3 Steps
An Intro to Server-Side Javascript
Assumptions: You have a text editor (like Atom) to create files. You know how to use the terminal. You have Node.js and NPM installed. You have cursory experience with Javascript.
This tutorial is going to help you create your first Hello World web server. Thread based web servers are a bit Let’s jump right into it:
- Open up your editor of choice and create a hello_world.js file containing this code:
var http = require(‘http’);
var server = http.createServer(function (req, res) { res.writeHead(200); res.end(‘Hello World’); });
server.listen(4000); console.log(‘Server Running on Port 4000’);
2. Open up your Terminal, cd into the directory where you saved your hello_world.js file and run this:
$ node hello_world.js
I’ll explain what’s happening here later.
3. Now point your browser to localhost:4000 and you should see your barebones Hello World server
For those more comfortable with the terminal can curl directly from it with:
$ curl localhost:4000
You’ll get the same Hello World response from the server.
What’s Really Happening Here
On the first line of your hello_world.js file, we added the HTTP module and assigned it the variable `http`. On the next line we create a variable called `http.createServer` with a closing argument passed to it for each time it gets an HTTP request. The `req` object contains data about the HTTP request that raised the event, while the `res` object sends it back to the client in response to whatever the `req` object reads. In this case we have it write the 200 header letting us know everything is OK with “Hello World” in the body.
Fun Fact: You might not know it, but you used Node’s module system here as well. When you required http, it included the core module of node’s HTTP API.
That’s it! Now experiment with your server and see if you can figure out how to require a non-core module.